Kotlin if-then expressions
The if construct in Kotlin works almost the same as in Java.
val theQuestion = "Doctor who?"
val answer = "Theta Sigma"
val correctAnswer = ""
if (answer == correctAnswer) {
println("You are correct")
}
Another example, this time, let’s use the else if
and else
clause.
val d = Date()
val c = Calendar.getInstance()
val day = c.get(Calendar.DAY_OF_WEEK)
if (day == 1) {
println("Today is Sunday")
}
else if (day == 2) {
println("Today is Monday")
}
else if ( day == 3) {
println("Today is Tuesday")
}
else {
println("Unknown")
}
So far, it looks a lot like how you would you do it in Java. Doesn’t it? What’s different in Kotlin is, the if
construct isn’t a statement, it’s an expression. Which means, you can assign the result of the if
expression to a variable. Like this
val theQuestion = "Doctor who"
val answer = "Theta Sigma"
val correctAnswer = ""
var message = if (answer == correctAnswer) {
"You are correct"
}
else{
"Try again"
}
If you have only one statement (each) on the if and else block, you can even shorten the code, like this
var message = if (answer == correctAnswer) "You are correct" else "Try again"
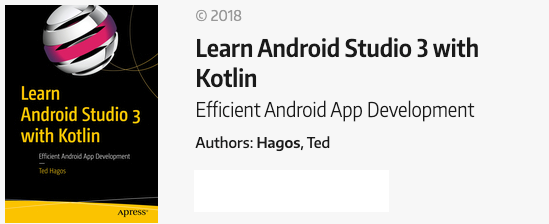
If you find any corrections in the page or article, the best place to log that is in the site's GitHub repo workingdev.net/issues. To start a discussion with me, the best place to do that is via twitter @lovescaffeine