How to create a simple Random number generator in Java
If you need to generate Random numbers in your Android app (assuming you’re using Java), you can simply use the java.util.Random
class.
Random random = new Random(); // (1)
mrandomnumber = random.nextInt(100); // (2)
(1) Creates a new Random object
(2) Creates a new random integers not greater than 100
Java’s Random class generates not only integers, it can also handle double, float, boolean etc., so, make sure you check out the docs at java.util.Random docs page
Here’s a small sample on how to use the Random class in Java. The RandomNumber class has two methods named getNumber()
and createRandomNumber()
, it’s supposed to behave like a Singleton so, it’s designed to create a random number only once.
import android.util.Log;
import java.util.Random;
public class RandomNumber {
private String TAG = getClass().getSimpleName();
int mrandomnumber;
boolean minitialized = false;
String getNumber() {
if(!minitialized) {
createRandomNumber();
}
Log.i(TAG, "RETURN Random number");
return mrandomnumber + "";
}
void createRandomNumber() {
Log.i(TAG, "CREATE NEW Random number");
Random random = new Random();
mrandomnumber = random.nextInt(100);
minitialized = true;
}
}
Then, you can use it like this from MainActivity
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
RandomNumber data = new RandomNumber();
((TextView) findViewById(R.id.txtrandom)).setText(data.getNumber());
}
}
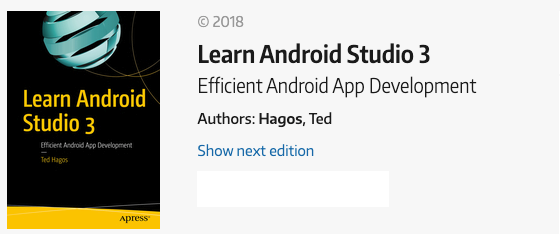
If you find any corrections in the page or article, the best place to log that is in the site's GitHub repo workingdev.net/issues. To start a discussion with me, the best place to do that is via twitter @lovescaffeine