How to open a file in Kotlin
Just like in Java, you use the java.io
classes, like this;
import java.io.FileNotFoundException
import java.io.FileReader
import java.io.IOException
fun main(args: Array<String>) {
var fileReader: FileReader
try {
fileReader = FileReader("README.txt")
var content = fileReader.read()
println(content)
}
catch (ffe: FileNotFoundException) {
println(ffe.message)
}
catch(ioe: IOException) {
println(ioe.message)
}
}
It can actually be much simpler than the above code; because in Kotlin, you don’t have to use try-catch if you don’t want to. Exception handling is optional. So, you write the code like this;
import java.io.FileReader
fun main(args: Array<String>) {
var fileReader = FileReader("README.txt")
var content = fileReader.read()
println(content)
}
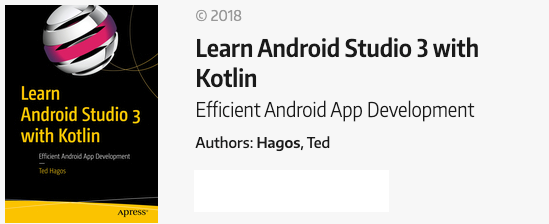
If you find any corrections in the page or article, the best place to log that is in the site's GitHub repo workingdev.net/issues. To start a discussion with me, the best place to do that is via twitter @lovescaffeine